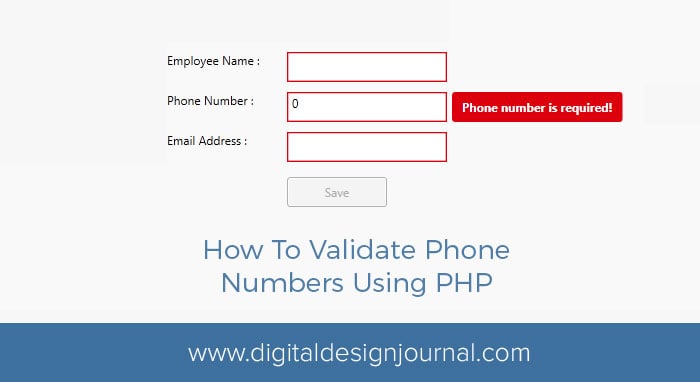
PHP is a very popular server-side programming language. One of the obvious task for a server-side programming language is to process forms. Forms are used to get data from website users. You could receive different inputs from what you expected due to mistakes of users. Also, hackers could use forms to access to your internal data. Hence, it is very important to validate user input data before using them for various purposes.
Phone numbers are an essential input field in many forms. There are two ways that you can use to validate the phone numbers in PHP. you can either use PHP inbuilt filters or regular expressions for that purpose.
Phone numbers are different from country to country. When you are creating a web application that will be used by people around the globe, you have to write codes which verify each of these requirements.
You might like this :
Phone Number Validation in PHP
Using inbuilt PHP filters easier. But, as there are different types of formats for phone numbers, you have to use regular expressions to validate these phone numbers. PHP provides you with several very powerful functions for parsing regular expressions.
Anyway, we will discuss both methods in this tutorial. First, we will study PHP filters.
Validating Phone Numbers with Filters
We don’t know what the user will enter in the input fields. There are some certain characters that we can expect in a phone number.
Those are digits, ‘-’, ‘.’, and ‘+’. The user sometimes could enter any other characters by mistake or with malicious intention.
We must remove those characters before doing any processing. PHP has built-in functions for this purpose. We call it sanitizing.
It will strip off any invalid characters in phone numbers.
This is how you’re going to do it.
function validating($phone){
$valid_number = filter_var($phone,FILTER_SANITIZE_NUMBER_INT);
echo $valid_number."
";
}
Code language: PHP (php)
You can see that we have used the filter_var
function with a FILTER_SANITIZE_NUMBER_INT
constant.
Let’s try this out with a few phone numbers.
function validating($phone){
$valid_number = filter_var($phone, FILTER_SANITIZE_NUMBER_INT);
echo $valid_number."
";
}
validating("202$555*0170");
Code language: PHP (php)
Output
2025550170
Great!
It strips off the $
sign and *
sign and returns only digits. Sometimes you may want to allow -
in phone numbers. For example, 202-555-0170 is a valid phone number. Let’s see how filter_var
function reacts to it.
function validating($phone){
$valid_number = filter_var($phone, FILTER_SANITIZE_NUMBER_INT);
echo $valid_number."
";
}
validating("202-555-0170");
Code language: PHP (php)
Output
202-555-0170
Great again! We get exactly what we want with filter_var
and FILTER_SANITIZE_NUMBER_INT
. There is one more thing. In international formatting, you need to allow the +
sign with the country code. We have to see if filter_var
supports the +
sign.
Let’s take +1-202-555-0170 and see what output filter_var
gives for it.
function validating($phone){
$valid_number = filter_var($phone, FILTER_SANITIZE_NUMBER_INT);
echo $valid_number."
";
}
validating("+1-202-555-0170");
Code language: PHP (php)
Output
+1-202-555-0170
Excellent.
There is still a small issue. We did not check the lengths of phone numbers yet.
Let’s check the number 2025550170000
.
function validating($phone){
$valid_number = filter_var($phone, FILTER_SANITIZE_NUMBER_INT);
echo $valid_number."
";
}
validating("2025550170000");
Code language: PHP (php)
Output
2025550170000
Well, filter_var
is not able to validate the length of a phone number. You may want to validate it manually. You can see that the length of a phone number, which includes a country code, could be between 10 and 14. Of course, this length is only valid if we remove ‘-’ in numbers. Let’s do it.
function validating($phone){
$valid_number = filter_var($phone, FILTER_SANITIZE_NUMBER_INT);
$valid_number = str_replace("-", "", $valid_number);
if (strlen($valid_number) < 10 || strlen($valid_number) > 14) {
echo "Invalid Number
";
} else {
echo "Valid Number
";
}
}
validating("+1-202-555-0170000");
Code language: PHP (php)
Output
Invalid Number
Code language: JavaScript (javascript)
That is our final code. It gives us the expected results. Next, we will see how do we validate phone numbers using Regular Expressions
Validating Phone Numbers with Regular Expressions
A lot of beginner-level developers find Regular Expressions difficult. Well, actually, learning regular expressions is easy. Using it requires good reasoning and logic. It gives great flexibility and power to developers. Therefore, Regular Expressions are such an important tool for any developer.
In the simplest form, the phone number is a 10 digits code without any other characters.
You use the following pattern to represent that. You can use '/^[0-9]{10}+$/'
in Regular Expressions to represent that.
PHP provides the preg_match
function to parse Regular Expressions.
Check the following code.
function validating($phone){
if(preg_match('/^[0-9]{10}+$/', $phone)) {
echo "Valid Email
";
}else{
echo "Invalid Email
";
}
}
Code language: PHP (php)
Let’s try this out now.
We will take several valid and invalid phone numbers and see whether our new code provides us with accurate results.
function validating($phone){
if(preg_match('/^[0-9]{10}+$/', $phone)) {
echo "Valid Email
";
}else{
echo "Invalid Email
";
}
}
validating("2025550170"); //10 digits valid phone number
validating("202555017000"); //12 digits invalid phone number
validating("202$555*01"); //10 letters phone number with invalid characters
validating("202$555*0170"); //10 digits phone numbers with invalid characters
Code language: PHP (php)
Output
Valid Email
Invalid Email
Invalid Email
Invalid Email
Great! We get results as we want it. You can see we have covered various possible inputs in the code.
Next, we will try to validate phone numbers with 202-555-0170
format to validate.
We will have to slightly change our regular expression for that.
function validating($phone){
if(preg_match('/^[0-9]{3}-[0-9]{3}-[0-9]{4}$/', $phone)) {
echo "Valid Email
";
}else{
echo "Invalid Email
";
}
}
validating("202-555-0170");
Code language: PHP (php)
Output
Valid Email
Notice that we have changed our regular expression to '/^[0-9]{3}-[0-9]{3}-[0-9]{4}$/
which will strictly look for the 000-000-0000
pattern.
Finally, let’s validate a phone number which has an international code.
Some countries have an international code with one number while the other countries include two numbers in their country codes.
So you should be able to take that into account when writing your regular expression.
function validating($phone){
if(preg_match('/^\+[0-9]{1,2}-[0-9]{3}-[0-9]{3}-[0-9]{4}$/', $phone)) {
echo "Valid Email
";
}else{
echo "Invalid Email
";
}
}
validating("+1-202-555-0170");
validating("+91-202-555-0170");
Code language: PHP (php)
Output
Valid Email
Valid Email
Conclusion
You can see how powerful Regular expressions are from these examples. You can validate any phone number against any format using Regular Expressions. On top of that PHP provides a very easy way to work with them. That’s it for Phone number validation with PHP. I will meet you with another tutorial.